đ iter - Awesome Go Library for Data Structures and Algorithms
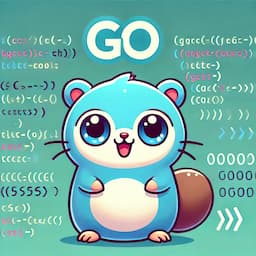
Go implementation of C++ STL iterators and algorithms.
Detailed Description of iter
iter
Note: I'm currently working on making it work with Go generics. For previous version, please check v1
branch.
Go implementation of C++ STL iterators and algorithms.
Less hand-written loops, more expressive code.
README translations: įŽäŊ䏿
Motivation
Although Go doesn't have generics, we deserve to have reuseable general algorithms. iter
helps improving Go code in several ways:
-
Some simple loops are unlikely to be wrong or inefficient, but calling algorithm instead will make the code more concise and easier to comprehend. Such as AllOf, FindIf, Accumulate.
-
Some algorithms are not complicated, but it is not easy to write them correctly. Reusing code makes them easier to reason for correctness. Such as Shuffle, Sample, Partition.
-
STL also includes some complicated algorithms that may take hours to make it correct. Implementing it manually is impractical. Such as NthElement, StablePartition, NextPermutation.
-
The implementation in the library contains some imperceptible performance optimizations. For instance, MinmaxElement is done by taking two elements at a time. In this way, the overall number of comparisons is significantly reduced.
There are alternative libraries have similar goals, such as gostl, gods and go-stp. What makes iter
unique is:
-
Non-intrusive. Instead of introducing new containers,
iter
tends to reuse existed containers in Go (slice, string, list.List, etc.) and use iterators to adapt them to algorithms. -
Full algorithms (>100). It includes almost all algorithms come before C++17. Check the Full List.
Examples
The examples are run with some function alias to make it simple. See example_test.go for the detail.
Print a list.List | |
---|---|
|
|
Reverse a string | |
|
|
In-place deduplicate (from SliceTricks, with minor change) | |
|
|
Sum all integers received from a channel | |
|
|
Remove consecutive spaces in a string | |
|
|
Collect N maximum elements from a channel | |
|
|
Print all permutations of ["a", "b", "c"] | |
|
|
Thanks
License
BSD 3-Clause